Create a fast auto-documented, maintainable and easy-to-use Python API in 5 lines of code with…
Perfect for (unexperienced) developers who just need a complete, working, fast and secure API
Create a fast auto-documented, maintainable and easy-to-use Python API in 5 lines of code with FastAPI (part 1)
You have a great python program that you want to make available to the world. With FastAPI you can speedily create a superfast API that’ll allow you to make your Python code available for other users.
In this article we’re going to create an API in 5 lines of code. What? Five lines?! That’s right; FastAPI isn’t called FastAPI because it is many times faster than frameworks like Django or Flask; it’s also super easy and fast to set up.
After we’ve created our initial API we’ll expand it the next part(s), demonstrating all the necessary bits of knowledge you need to create a fully functional, superfast, production-ready API. Let’s code!
Introduction to FastAPI
Let’s first a really quick look at our framework. FastAPI is a modern, open-source, fast, and highly performant Python web framework used for building Web APIs. Applications running under Uvicorn rank as one of the fastest Python frameworks available, only below Starlette and Uvicorn themselves (wich are used internally by FastAPI). Sounds good right? Let’s waste no more words and see how to use it.
Installation
Installation is pretty simple. We’ll first install FastAPI with PIP. Then we also need to install Uvicorn. This is the web server on which FastAPI runs.pip install fastapi uvicorn
It is recommended to install Python dependencies in a virtual environment. This way you keep your projects disentangled. Check out this article for simple instructions on how to use a virtual environment.
Creating our API
All necessary tools are installed, let’s create our API with a single route at the root in five lines. We’ll create a file called main.py
: this file will be the main entrypoint for our app and will define our API. Insert the code below:from fastapi import FastAPI
app = FastAPI()
@app.get("/")
def simple_route():
return {"Hello": "There"}
See how easy this reads? We’re importing fastapi
, creating a new instance that we’ll store in the app
variable. That’s our API defined already! In the last three lines we define a route. API created!
Starting up our API
Time to spin up our API. Open a terminal and navigate to the folder where the main.py
file from the previous step is located (check out this article if you’re unexperienced with using a terminal). Execute the following command:uvicorn main:app --reload
This calls Uvicorn and tells it to run the app
(the variable defined in the previous step) that’s located in the main.py
file. The --reload
flag reloads if we save changes in order to speed up development; we don’t have to stop and start the API manually in order to see changes.
Make sure to activate your virtual environment first if you use one. Another way to call uvicorn is python -m uvicorn main:app --reload
. This does exactly the same, only you can specify a specific python.exe eg:c:/mike/myProj/venv/scripts/python.exe -m uvicorn main:app --reload
Testing our route
If all is well you’ll see ‘application startup complete’. If you don’t specify otherwise then uvicorn runs on your localhost, on port 8000. So open a browser and to to localhost:8000
. You should see the following:
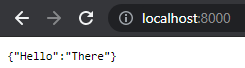
Autodocumentation
One of my favorite features about using FastAPI is that it automatically generates instructions and an overview on how to use the API; which routes are there, examples how to call and you can even test routes and see the return values! Check this out at localhost:8000/docs
or localhost:8000/redoc
. These are different styles for the same documentation.
Conclusion
In about 5 minutes we’ve spun up a simple API with just one route on the root. but there’s not much interactivity yet. We want to connect our API so it should be able to receive messages and send some too!
In the next part we’ll check out how we can receive query parameters and a body. Also we’ll create more routes within the API and organize these routes in a smart way. Later we’ll focus on data validation, security and deployment using Docker.
If you have suggestions/clarifications please comment so I can improve this article. In the meantime, check out my other articles on all kinds of programming-related topics.
Happy coding!
— Mike
P.S: like what I’m doing? Follow me!