Create a fully fledged, professional API with Flask in Python — Part 1
Professionally and securely allow access to your Python programs in 6 steps
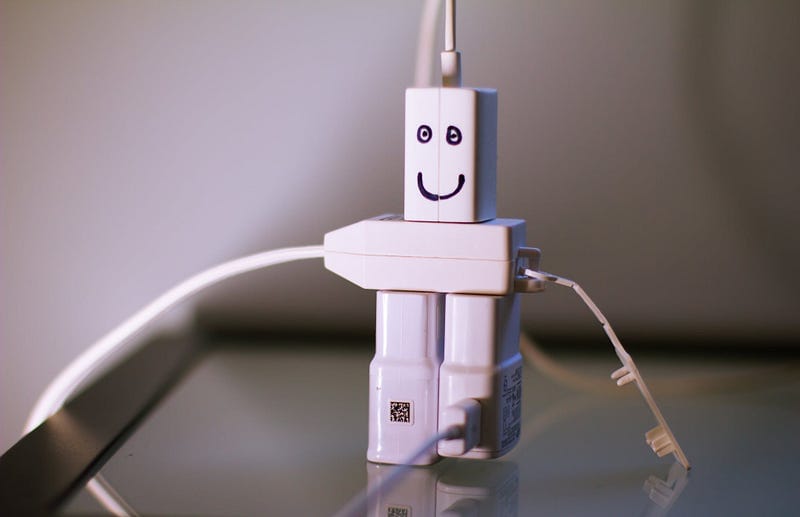
Professionally and securely allow access to your Python programs — setting up the basics in 6 easy steps
An application without an API is much like a restaurant without waiters. In restaurants you don’t have to communicate with the kitchen and wait for your order to enjoy a meal. In the same way a website doesn’t have to know how to communicate with your database for example. Like a waiter, our API receives an order from our website: ‘I’d like some user statistics with a side of meta data”. The API then checks the order (are you allowed to have that data?), convinces our database (the chef) to cook him up some tasty information, waits for the database to finish and finally returns the data to the website.
Why would you need an API?
An API allows two (or more) services to talk to each other in a decoupled way. The most common example is an API that handles communication between a website and a database. It provides access, convenience, security and extra functionalities. Let’s take a look at look a short example of each of these points.
1. Access
Imagine you’ve created a Python program called pixelcounter.py. It reads an image file and counts the number of pixels. At the moment you put your image file on a USB, walk to the computer that pixelcounter.py is on, put the image on the computer and load it up in pixelcounter.py to finally start counting.
This works, only thing is: we’re programmers. We don’t have time to put stuff on USB sticks, let alone walk to something other than the coffee machine! A nice solution would be to put the API and pixelcounter.py on a server. That way people can send images to be counted.. Easy!
2. Convenience
API’s also provide a lot of convenience. In order to send an email just send the receiver, subject and message to an API. You don’t need to understand how and SMTP works if you can just send your data to the API.
3. Extra functionality
Using an API can also provide extra functionality. One example is that you can count which user requested what data. That way you can limit usage, check out which functionalities people are most interested in, what functionalities don’t get used at all or charge users per request.
4. Security
An API allows two programs to talk to each other in a secure way. For a website like Facebook to retrieves your friends it needs to get that data from a database. You don’t want another person to get your personal data. That’s where an API comes in; it checks who makes the request and whether that person is allowed to get that data.
How to create an API?
In this article we’ll start creating an API that’ll allows us to do all the thing described above. In this part we’ll focus on setting up our project, getting some basic functionality working and testing our routes. If you see any terms you’re unfamiliar with, check out the glossary at the bottom of this page.
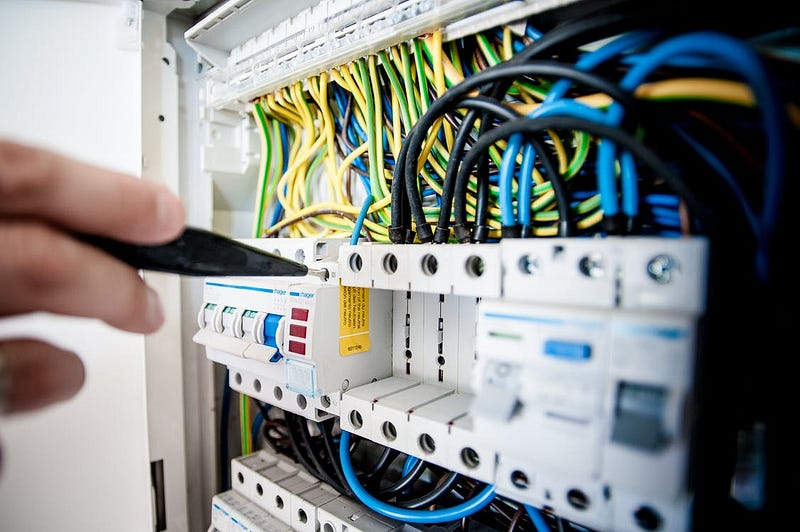
Follow along these steps to create a project with all the necessary dependencies, Then we’ll create a controller that will handle requests on a route.
Step 1. Create your python project
Create your root folder: the folder that will contain the project. In this example we’ll use c:/my_root_folder
Step 1.1 (OPTIONAL) Create a virtual environment
Creating a virtual environment can help you keep your installed packages in a neat bundle. More information about understanding and creating venvs in this article. Here’s how to do it for this proejct:
Navigate to your root folder and execute this code. python -m venv c:/my_root_folder/venv
This will create a folder that will house all of your installed packages. Don’t forget:
- activate your environment before installing packages by navigating to
c:/my_root_folder/venv/scripts
and executingactivate
. Now you are in your environment and can install packages in here - execute your script by calling
c:/my_root_folder/venv/Scripts/python.exe
. We’ll get into it more later
Step 2. Install dependencies
We’re going to need two packages so we’ll need to install Flask and flask-restful. pip install Flask flask-restful
Step 3. Structure your project
Next step: create some folders in which we can put our different parts of te API, namely our config, routes and controllers: mkdir config routes controllers
Step 4. Create the API
Our project is set up, time to build our API! create a file called main.py in our root folder and give it the following contentfrom flask import Flask
from flask_restful import Apiapp = Flask(__name__)
api = Api(app)if __name__ == "__main__":
# start up api
app.run(port=5000, debug=True)
What’s happening here?:
This script imports all the required packages and instantiates an app and api. It then runs the app on localhost port 5000. Execute your script:c:/my_root_folder/venv/Scripts/python.exe c:/my_root_folder/main.py`
You’ll notice that your script is running but is not stopping. The server we’ve created is continuously listening for requests. Lets give it something to listen to.
Step 5. Create a route and a controller
We’d like to go to localhost:5000/test and have the API do something when we navigate to that page or POST something to that location. In order to to this we need our API to listen to a route, namely /test.from flask import Flask
from flask_restful import Api
from controllers.testController import TestControllerapp = Flask(__name__)
api = Api(app)
api.add_resource(TestController, '/test')if __name__ == "__main__":
# start up api
app.run(port=5000, debug=True)
The code above is almost identical to the first piece of code. As you can see it’s easy to listen to a route. This happens in line 7: api.add_resource(TestController, ‘/test’)
It listens to requests at /test and passes the request to our TestController, which is a class that we import on line 3. Let’s create this controller. Create c:/my_root_folder/controllers/testController.py
with this content:from flask_restful import Resource, requestclass TestController(Resource):
def get(self):
return {
"message": "get: hello from the testcontroller"
}
There are multiple ways of requesting data from an API. You can send GET request of POST requests. A GET is for retrieving data, like retrieving my profile picture. POST requests are for submitting data like submitting a comment. There are many more types, which we’ll get into in the next part. First let’s test a simple API.
The TestController above implements a get method that handles get request that has been passed to the controller. Right now we’ll just return a simple message.
Step 6. Testing
Open a browser and navigate to localhost:5000/test. If all went well you’ll see the message we’ve define in our controller.
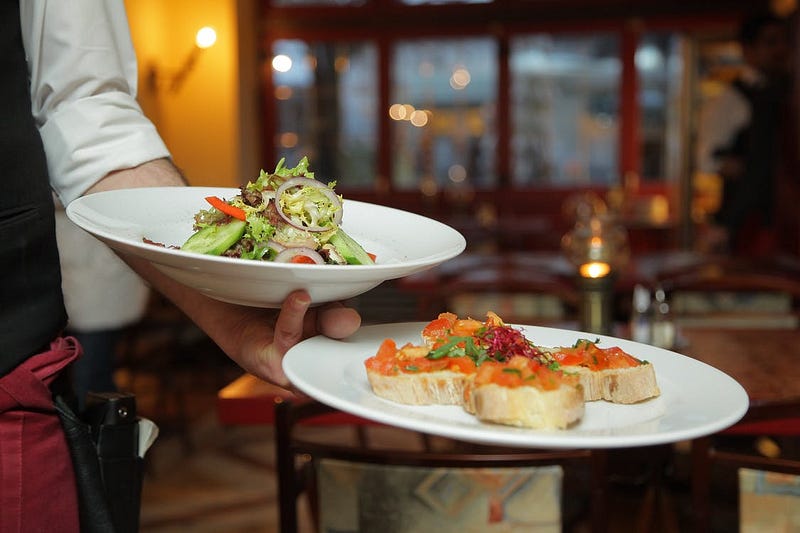
Conclusion
Congratulations on creating your first API! Our new API is up and running but it’s pretty dumb. In the next part we’ll implement some more functionalities like different types of request, security with JSON web tokens and a connection to a database. Follow me to stay tuned!
— Mike
Glossary
Some terms, explained
- API = Application Programming Interface. Allows you to connect two programs
- request = A command you send to an API. You’re requesting it to do something
- response = Something the API gives back; data, an error or a status
- route = A path on a website. It’s the bold part in www.medium.com/@mikehuls or test.com/this/is/a/path
- status code = A code that represents a stat of the api. Everybody has heard of 404: not found!