Create and publish your own Python package
A short and simple guide on how to pip install your custom made package
You are probably familiar with requests, Pandas, Numpy and many other packages that you can install with pip. Now it’s time to create your own package! In this article we’ll go through the required steps to package and publish your Python code for the whole world to pip install. First we’ll look at packaging your code, then how we can publish it to make it accessible.
(Would you rather share your code with just a select few people like coworkers? It’s also possible to allow people to pip install a package from a private repository. This even works when including your package in a Docker container!)
Goal and preparation
For this article, I’ve created a few truly essential functions that I’d like to share with the world. Let’s create a package called “mikes_toolbox” that people can use by simply pip install mikes_toolbox
.
First we’ll sketch an overview of how the installation process works: if someone calls pip install mikes_toolbox
the code has to come from somewhere. Pip searches for a package with that name on PyPi; the Python Package Index. You can think of this as a YouTube for Python packages. First we’ll package our code and then upload it to PyPi so that others can find our package, download it and install it.
Packaging
First we’ll package our code. We’ll create a project directory called ‘toolbox_project’ that contains the following directories and files:toolbox_project
mikes_toolbox
__init__.py
functions.py
decorators.py
LICENSE.txt
README.md
setup.cfg
setup.py
The content of the project folder consists of two parts: the mikes_toolbox folder and 4 additional files. The folder is our actual package that contains our source code. The 4 additional files contain information on how to install the package and some additional information.
Package folder
mikes_toolbox is our actual package and contains all of our source code. Make sure to name this folder the same as your desired package name. In my case it has this content:
- mikes_toolbox/function.py and decorators.py
This is my source code. Function.py contains a function called weirdCase() for example; a function that’ll turn a string completely unreadable. - mikes_toolbox/__init__.py
This file is required. It tells python that mikes_toolbox is a Python package folder. You can keep it empty. Optionally you can include import statements here to make importing code from your package easier. An example:
Includefrom functions import weirdCase
. This way people don’t have tofrom mikes_toolbox.functions import weirdCase
once the package is installed, instead they can justfrom mikes_toolbox import weirdCase
.
LICENSE.txt
Describes how people can use your license. Just select one from this site and paste the contents in this file.
README.md
This file contains information about the package: what does it do? What are its features? How to install and use it? Check out an example here or here.
This file is written in markup. Check out this site for an editor that already contains some example markup; just edit it to fit your needs.
setup.cfg
This is a simple file that just contains the code below. It refers to the README.md.[metadata]
description-file = README.md
setup.py
This file makes sure the package installs correctly. Copy the code below and modify it where needed. Most entries are logical but the download_url needs a little explanation (see below):
download_url
When you pip install pandas
pip searches for pandas on PyPi. Once found, PyPi tells pip where it can download the package. Most of the time this is a file on a git repository. The download_url keyword refers to the location of this file.
In order for us to get a URL we first have put our source code somewhere that pip can reach. The best way to do this is to upload your code to a GitHub repo and create a release. This process is super-easy, here are the steps:
- Create a repository (name doesn’t have to match package name but it is more orderly).
- In your repo, on the right side; click “create a new release”
- Fill in “tag version” to be the same as in setup.py (version keyword)
- Give the release a title and description. This is not reflected in the package, this is purely for keeping an overview in your repo
- Click “publish release”
- Copy the link address of “Source Code (tar.gz)
- Paste the copied link address as the value op the download_url in setup.py
That’s it! Your package is now downloadable and installable. The next step is to take care of distribution.
Distributing
The only thing we’ve done so far is packaged our code and uploaded it to GitHub, pip still has no idea our package exists. So let’s make sure pip can find our package so that people can install it.
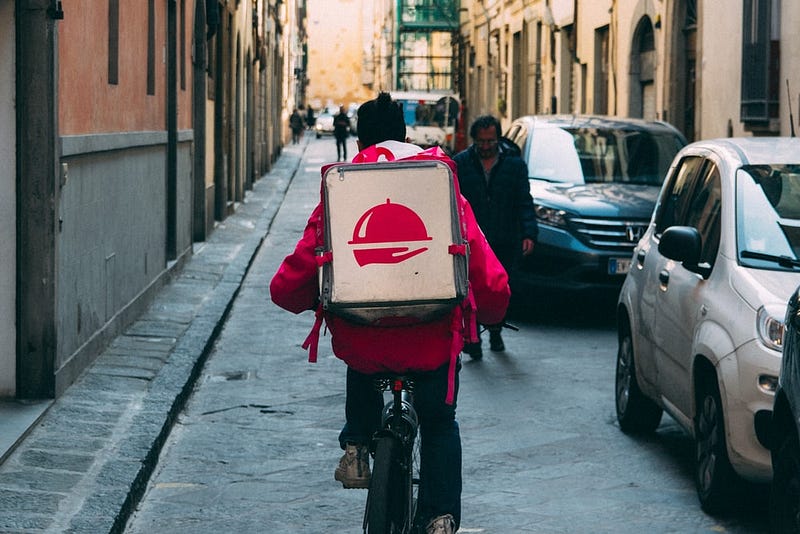
PyPi account
If you want to post video’s to YouTube you need to create an account first. If you want to upload packages to PyPi you also need to create an account first. Go to PyPi and register an account. Confirm your email address before continuing.
Creating a source distribution
This creates a tar.gz file that contains everything needed to run the package. Open a terminal, cd to your project directory and execute the command below:
python setup.py sdist
(Unfamiliar with the terminal? Check out this article for the absolute basics)
Uploading
We’re ready to upload our package! First we need to pip install Twine which’ll help us upload. Simply pip install twine
.
The last step is to actually upload the package. In a terminal, cd to your project directory if you’re not there already and executepython -m twine upload dist/*
Twine will ask you for your PyPi credential but after that your package should be uploaded!
Testing
Create a new python project and (optionally) spin up a new virtual environment. Then pip install mikes_toolbox
. Test the code by callingfrom mikes_toolbox import weirdCase
print(weirdCase("This function is essential and very important"))
Updating your package
If you update your package you need to make sure to update the version in setup.py and create a new release with the same tag on GitHub. Also update the download_url in setup.py.
Once you’ve done this users can update your package usingpip install mikes_toolbox --upgrade
Conclusion
In these easy steps you’ve learnt how to package your code and publish it to the world using PyPi and pip. In addition you’ve created a central place where your code lives which can be uses for tracking bugs, posting issues or requesting new features. No more sharing code via mail
I hope I’ve clarified a lot of the process of creating and distributing Python packages. If you have suggestions/clarifications please comment so I can improve this article. In the meantime, check out my other articles on all kinds of programming-related topics. Happy coding!
— Mike
P.S: Like what I’m doing? Follow me!
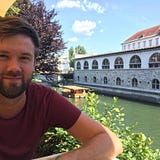