Python — Print colored text with ease
How to Console.log, console.warn and console.error in Python
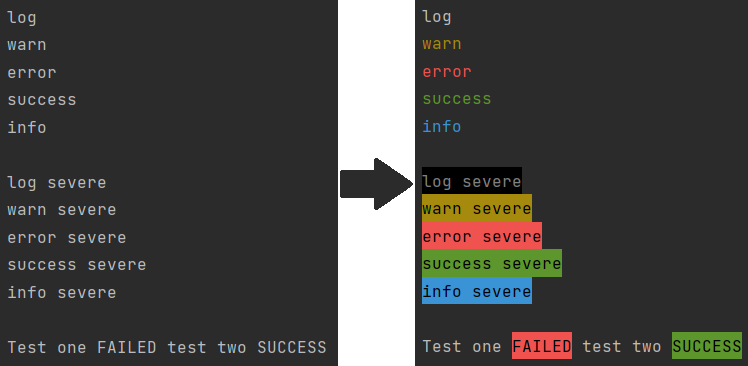
Which of the two screenshot in the image above looks better? I’d definitely choose the right one! The colors immediately draw your eye to important things and give you extra information that you can see at a glance.
This article touches two subjects: first we’ll get into how printing color in the terminal works on a
- how printing colors in the terminal works
- Py-console; a Python package that allows you to print colored outputs easily
At the end of this article you’ll be able to print colored outputs so what are we waiting for? Let’s code!
1. Understanding printing
First we’ll check out what the terminal needs to print colored text. This’ll show you how printing colored text works on the lowest level. In the next parts we’ll replace this approach by something that’s far easier to work with.
When execute the print
function in Python the text appears in a terminal. Our goal is to add some data to this value so that our terminal knows to add some color when displaying the text. These pieces of extra data are ANSI escape character sequences
that are used to control cursor location, color and font styling e.g. in terminals. The terminal interprets these sequences as commands, rather than text to just display.
In the example below we use Python to tell the terminal to print the text ‘This is our message’ with white text and a yellow background:print('\x1b[0;39;43m' + 'This is our message' + '\x1b[0m')
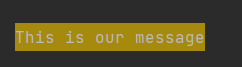
The first piece of ANSI code (\x1b[0;39;43m
) is a command for the terminal to color all subsequent text in accordance with the specified parameters. We can specify the style, text color and background color with integers in the 0;39;43
part. The second piece of ANSI code at the end(\x1b[0m
) tells the terminal to reset the color options so that not all subsequent text is colored white and yellow anymore.
The problem with this approach is that this approach is not uniform per platform; ANSI code works on Unix and Macs but are unsupported on Windows. Also we don’t want to type out these specific, hard to understand pieces of code. Let’s find a simpler, cross-platform approach!
2. Py-console; easily print colors in Python
Py-console is a package that I’ve created that makes printing colors super-easy. It emulates JavaScripts well-known methods likeconsole.log
, console.warn
, console.error
etc. It also adds some more functionalities.
Want to create your own Python package? → check out this article for instructions. Also check out this article for creating your own private package)
Installation
First we’ll install py-console:pip install py-console
Log, warn, error, success, info
After installing we can simply call one of 5 functions that will print the text in a specified color:from py_console import consoleconsole.log("log")
console.warn("warn")
console.error("error")
console.success("success")
console.info("info")
This produces:
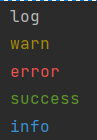
Toggle time and setting the timestamp
We can also add a timestamp to out outputs by specifying this for the console or per method:console.setShowTimeDefault(True)
or
console.log('log', showTime=True)
These will add the time in front of the string you want to print out:
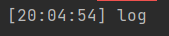
In addition you can also set the timeformat. Check out more information on this here. The example below sets the time format to include years, months and days.console.setTimeFormat('%y-%m-%d %H:%M:%s')
Severer warnings
In the methods we can specify a ‘severe’ flag so that we have more clear outputs, reversing the text color and background color:from py_console import consoleconsole.log("log", severe=True)
console.warn("warn", severe=True)
console.error("error, severe=True")
console.success("success, severe=True")
console.info("info, severe=True")
This will produce the output below:
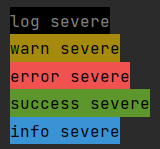
Highlighting
Lastly we can also highlight certain parts of a string. Check out this example where we use the console.warn function:

Conclusion
In this article we’ve focused briefly on how a terminal prints colored text to display important information more clearly. Then we’ve looked at py-console and how it can make colored output very accessible.
I hope that I’ve shed some light on how the terminal can be instructed to print colors and I hope I’ve left you with a nice package for creating beautiful console outputs. If you have suggestions/clarifications please comment so I can improve this article.
In the meantime, check out my other articles on all kinds of programming-related topics like these:
- Create a fast auto-documented, maintainable and easy-to-use Python API in 5 lines of code with FastAPI
- Python to SQL — UPSERT Safely, Easily and Fast
- Create and publish your own Python package
- Create Your Custom, private Python Package That You Can PIP Install From Your Git Repository
- Virtual environments for absolute beginners — what is it and how to create one (+ examples)
- Dramatically improve your database insert speed with a simple upgrade
- Read more about py-console here and more about colorama here.
Happy coding!
— Mike
P.S: like what I’m doing? Follow me!